Contributing
Code of Conduct
Be goodโข๏ธ.
TODO: more info
Development + Contributing: Getting Started
Prerequisites
bash
- On Windows, you can get this via cygwin or
git
(which also installs cygwin)
- On Windows, you can get this via cygwin or
- Recommended: Volta
- Please consider the installation chapter for pros, cons and installation instructions.
yarn@1
Node@16
Setup
git clone https://github.com/Domiii/dbux.git
cd dbux
code dbux.code-workspace # open project in vscode
yarn install && yarn i # install dependencies
Finally, you might want to enable Dbux auto start by default:
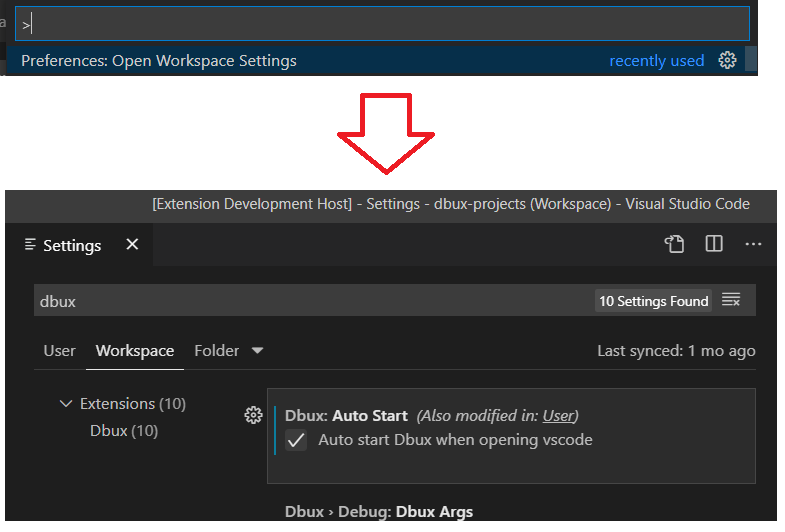
If dependencies bug out, sometimes running the (very aggressive) clean-up command can help: npm run dbux-reinstall
.
Of course, we don't recommend this, unless absolutely necessary. It will delete your yarn.lock
file, which can lead to installation of package versions that have breaking changes in them, potentially introducing even more problems.
Start Development
- Open project + start build+watch mode
code dbux.code-workspace # open project in VSCode
yarn start # start webpack development build of all projects in watch mode - Go to your VSCode debug tab, select the
dbux-code
configuration and press F5. This runs the Dbux VSCode Extension in debug mode. - Inside the new window, you can then use Dbux VSCode Extension as usual (but in debug mode).
Contribute to Docs
We use docusaurus
for documentation.
- Follow the Setup steps.
- Run
code dbux.code-workspace
to open the Dbux project in VSCode - You can find all docs in the
docs_site
folder. Thedocs_site/content
folder houses most of the content source. - Run
yarn docs
to build + serve the docs locally (inwatch
mode), and test your changes. - Once you are done: run
yarn docs:build
(which does a few more pre-deployment checks) - If
yarn build
succeeds, feel free to send out a PR.
Build + Deploy docs
Inside of the docs_site
folder:
yarn build
- -> Make sure, there are no build problems.
yarn serve
- This serves the production build locally to allow you manually test and check whether the documentation site works correctly.
- You especially want to do this when introducing new complex logic or components.
yarn deploy
- Make the changes go live.
Documentation: Related Issues
Joining the Community
While you can certainly try to get started on your own, you probably make your life a lot easier by join the dev team on Discord first :)
Use Dbux Dev Build on Local Projects
When you want to debug a local project with a local Dbux dev build, we recommend using yalc
:
- Make sure you have compatible
npm
and/oryarn
versions, as explained here. - In your local Dbux folder:
- run
yarn yalc
- run
- Inside your target project folder:
yalc add --dev @dbux/babel-plugin @dbux/runtime @dbux/cli @dbux/common @dbux/common-node @dbux/babel-register-fork
- Add to .gitignore:
.yalc
yalc.lock
- NOTE: Sometimes (but rarely), the build cache needs to be flushed.
- E.g. when using
webpack
andbabel
:rm -rf ./node_modules/.cache/babel-loader
- E.g. when using
caution
This will not work (smoothly) with yarn@1
.
While generally linking the local dev build using yalc
works, it will not be able to install dependencies, and in general, yarn add
and yarn install
will always fail from now on, if you use yarn@1
. This is a well-known bug that was fixed for yarn@>=2
.
Hackfix workaround for yarn@1
:
- After
yalc add...
:- (If you use
yarn workspaces
: remove linkage to other workspace packages.) - Use
npm install
once, then removepackage-lock.json
. - (Bring back other workspace packages if needed.)
- If you use webpack or other bundlers, make sure,
.yalc
folder is in yourresolve.modules
(or other bundler's equivalent).
- (If you use
- Whenever you need to change things with
yarn add/remove
, first removeyalc
packages, then bring them back:yalc remove --all
yalc add ...
PROBLEM: (one of many) since npm install
ignores your yarn.lock
, and also might undo (some) deduplication, your dependencies might get real messy real fast. That is especially due to libraries often not following the convention of only introducing breaking changes with major releases. Meaning that all libraries that were added with (usual default of) caret (^) can potentially break due to this.
More References
Test the Production Build
Before submitting a PR, make sure to test it in prod
as well. This is important, especially if your changes are related to the parts of Dbux that juggle projects, file paths and/or system dependencies:
- Start production build (in
watch
mode):yarn start:prod
- IMPORTANT: Make sure only one build/watch mode is running at the same time.
- Install (and re-install) local build locally:
yarn code:install
- Uninstall:
yarn code:uninstall
- Uninstall:
- Incremental update:
yarn code:copy
, thenReload Window
(VSCode command)- NOTE: This copies all built
.js
files to the installed extension folder. - NOTE2: This is to save yourself time. You don't want to re-install the extension every time you make a change.
- NOTE: This copies all built
Deployment
If you have deployment privileges:
- Build production build and publish to
npm
andVSCode
Marketplace:yarn marketplace
- IMPORTANT: again, make sure, only one build/watch mode is running at the same time.
yarn pub
if you only want to publish tonpm
(not the marketplace)
More Unsorted Notes
Adding dependencies
We use Lerna with Yarn workspaces, so if you need to install a new package, instead of npm install pkg
, we do the following:
- Adding
pkg
to@dbux/something
(wheredbux-something
is the folder containing the package@dbux/something
):npx lerna add --scope=@dbux/something pkg
npx lerna add --scope=@dbux/common pkg
npx lerna add --scope=dbux-code pkg # note: dbux-code's package name has a dash (-), not a slash (/)!
- Adding
pkg
to the root'sdevDependencies
:yarn add --dev -W pkg
VSCode extension development
This section holds a few basics of VSCode extension development.
Adding a command/button
NOTE: VSCode's buttons are representation of commands, you must add a command before adding a button.
Register command in
contributes.commands
section of package.json as following(title and icon are optional):{
"commands": [
{
"command": "<COMMAND_ID>",
"title": "<TITLE>",
"icon": {
"light": "<PATH_TO_YOUR_ICON>",
"dark": "<PATH_TO_YOUR_ICON>"
}
}
]
}Bind the command with a callback function in js file:
import { registerCommand } from './commands/commandUtil';
registerCommand(context, '<COMMAND_ID>', callback);Depends on whether the command should be shown in command palette, add the following to
contributes.menus.commandPalette
section in package.json: Show command in command palette (use"when": "!dbux.context.activated"
instead if you want show it before dbux has been activated){
"commantPalette": [
{
"command": "<COMMAND_ID>",
"when": "dbux.context.activated"
}
]
}Hide command from command palette
{
"commantPalette": [
{
"command": "<COMMAND_ID>",
"when": "false"
}
]
}Finally, configure where the button should be shown (skip this step if you don't want a button): To show button in...
- navigation bar(upper-right corner), add the following to
contributes.menus.editor/title
{
"editor/title": [
{
"command": "<COMMAND_ID>",
"when": "dbux.context.activated",
"group": "navigation"
}
]
}- tree view title, add the following to
contributes.menus.view/title
(<VIEW_ID>
is defined incontributes.views.dbuxViewContainer
section)
{
"view/title": [
{
"command": "<COMMAND_ID>",
"when": "view == <VIEW_ID>",
"group": "navigation"
}
]
}- tree view item, add the following to
contributes.menus.view/item/context
(<VIEW_ID>
is defined incontributes.views.dbuxViewContainer
section and<NODE_CONTEXT_ID>
is defined programmatically inTreeItem.contextValue
)
{
"view/item/context": [
{
"command": "<COMMAND_ID>",
"when": "view == <VIEW_ID> && viewItem == <NODE_CONTEXT_ID>",
"group": "inline"
}
]
}- navigation bar(upper-right corner), add the following to
Some more Notes:
- You can sort buttons by adding suffix
@<number>
togroup
property. e.g."group": "inline@5"
- If you remove
"group": "navigation"
, the button will be listed in a dropdown menu, see Sorting of groups for more information - The
when
property defines when should the button be visible, see 'when' clause contexts for more available condition
- You can sort buttons by adding suffix
How to use Dbux on Dbux?
TODO: we have not fully added support for this yet.